If you are a developer and would like to add a new functionality or enhance a website, creating a plugin can be a great way. In this article, I will guide you how to create a WordPress plugin from scratch [step by step guide].
Basics
At first we will talk about the basics and then I will show you an example plugin.
Go to wp-content/plugins and create a directory insert-adsense-ad. In this example I will show you how to insert Adsense ad between posts.
As this is going to be a simple plugin we will not add any JS or CSS. But if you want you can create two directories js and css in your plugin root directory. After that you can create a JS or CSS files inside their respective directories and later inject them in the plugin.
Note: check at the end of this article, I have added how to inject js and css into your plugin.
Next is to create a PHP file, we will name it plugin.php. There is no restriction, you can name it anything you want.
Inside plugin.php, paste or type the following.
<?php
/**
Plugin Name: Insert Adsense Ad
Description: Inserts an ad code after every 2 posts
Version: 1.0.0
Author: M M Arif
Author URI: https://najigram.com
*/
Plugin Name = Name of the plugin which you will see in WordPress plugins page
Description = Short description of the plugin
Version = Version of the plugin for WordPress to recognize an update
Author = Creator of the plugin
Author URI = URL of the author
That’s pretty much it for the basics.
Ad code example
Our plugin is ready and WordPress will recognize and show it in the plugins page and even you can enable it, but it will do nothing for now.
Add the following code to plugin.php
which will add an ad to posts loop after every 2nd post.
function insert_ad_between_posts() {
global $wp_query;
if ( is_home() && ! is_admin() && $wp_query->is_main_query() && ( $wp_query->current_post % 3 == 2 ) ) {
echo '<div class="ad-code">YOUR AD CODE HERE</div>';
}
}
add_action( 'the_post', 'insert_ad_between_posts' );
Save and check your posts page now, you will see YOUR AD CODE HERE.
Full code for plugin.php:
<?php
/**
Plugin Name: Insert Adsense Ad
Description: Inserts an ad code after every 2 posts
Version: 1.0.0
Author: M M Arif
Author URI: https://najigram.com
*/
function insert_ad_between_posts() {
global $wp_query;
if ( is_home() && ! is_admin() && $wp_query->is_main_query() && ( $wp_query->current_post % 3 == 2 ) ) {
echo '<div class="ad-code">YOUR AD CODE HERE</div>';
}
}
add_action( 'the_post', 'insert_ad_between_posts' );
Inject JS and CSS
You can inject JS code with the following function. We have a js directory and inside should have a plugin.js file.
function ad_scripts() {
wp_enqueue_script( 'ad_script_identifier', plugins_url( '/js/plugin.js', __FILE__ ), array(), '1.0.0', true );
}
add_action( 'wp_enqueue_scripts', 'ad_scripts' );
Keep in mind that 1.0.0 is for the js file version, not the plugin. true
is for loading the file in the footer. The array()
is empty, but you can let’s say add jquery, which this script will be dependent on. It will become, array('jquery')
.
The CSS injection function should look like below:
function ad_styles() {
wp_enqueue_style( 'ad_styles', plugins_url( 'css/style.css', __FILE__ ) );
}
add_action( 'wp_enqueue_scripts', 'ad_styles' );
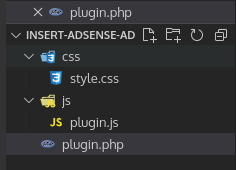
Full code
Here is the full code:
<?php
/**
Plugin Name: Insert Adsense Ad
Description: Inserts an ad code after every 2 posts
Version: 1.0.0
Author: M M Arif
Author URI: https://najigram.com
*/
function ad_scripts() {
wp_enqueue_script( 'ad_script_identifier', plugins_url( '/js/plugin.js', __FILE__ ), array(), '1.0.0', true );
}
add_action( 'wp_enqueue_scripts', 'ad_scripts' );
function ad_styles() {
wp_enqueue_style( 'ad_styles', plugins_url( 'css/style.css', __FILE__ ) );
}
add_action( 'wp_enqueue_scripts', 'ad_styles' );
function insert_ad_between_posts() {
global $wp_query;
if ( is_home() && ! is_admin() && $wp_query->is_main_query() && ( $wp_query->current_post % 3 == 2 ) ) {
echo '<div class="ad-code">YOUR AD CODE HERE</div>';
}
}
add_action( 'the_post', 'insert_ad_between_posts' );